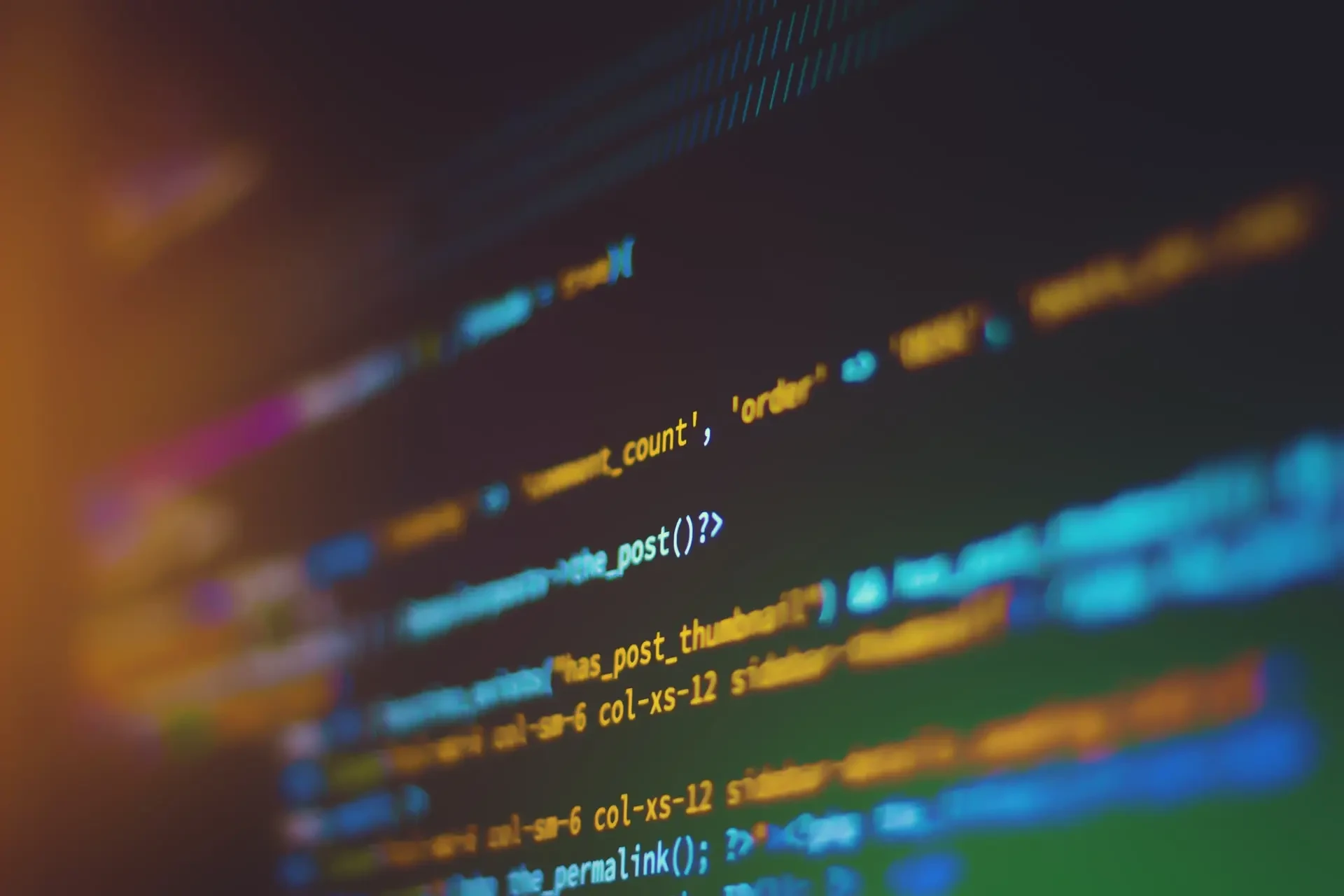
Code Review: 3 Common Mistakes of Junior developers
Code review is a way to share knowledge and convey experiences. In doing code review we often look at common mistakes that our fellow developer makes. Let’s take a look at common mistakes we see in code review.
1. Hardcoded String
A hardcoded string is a literal string. We often use hardcoded strings in our API request and even in our switch / if statements.
It might be easy to read but what if you suddenly misspelled the string, it will be hard to spot and debug.
Imagine if those strings were used multiple times in the file and suddenly you have to update all of it.
You will spend more time changing all of those literal strings.
Not to mention if you misspelled one. You will have to manually look for the misspelled string, costing more time.
const sampleFunction = (conditionalString: string) => {
switch (conditionalString) {
case'string1':
return'returning string1'
case'string2':
return'returning string2'
default:
return'returning string3'
}
}
const sampleFunction = (conditionalString: string) => {
if("string1" === conditionalString){
return'returning string1'
}
if("string2" === conditionalString){
return'returning string2'
}
return'returning string3'
}
}
const getData = fetchData("stringhere")
const getData2 = fetchData("stringhere")
How to resolve this?
Rather than using hardcoded strings. A better way is to store it in a variable. Especially if you reuse
the same string in the file. In a situation where you have to update the string. You only need to update the variable that contains the string.
const apiString = "stringhere"
const getData = fetchData(apiString)
const getData2 = fetchData(apiString)
As for a group of strings you can use enumeration for them.
enum STRING_SAMPLE {
STRING1 = 'string1',
STRING2 = 'string2',
DEFAULT = 'string3'
}
const sampleFunction = (conditionalString: string) => {
switch (conditionalString) {
case STRING_SAMPLE.STRING1:
return`returning ${STRING_SAMPLE.STRING1}`
case'string2':
return`returning ${STRING_SAMPLE.STRING2}`
default:
return`returning ${STRING_SAMPLE.DEFAULT}`
}
}
2. Functions for all
A function is a collection of codes that does a certain thing. Creating a function usually helps in chunking down our code into small pieces that performs a certain task. Aiding programmers to not rewrite their code over and over again.
A common misconception about functions is that they should be minimized at all cost. But in reality functions should only perform simple tasks rather than having an overloaded function that does everything.
A problem arises when a function does more than it should. Having a specific function that does a particular task is easier to read and understand than a function that does more than it should.
Don’t over complicate things.
Remember the “Simplicity is King”
3. Band-aid fix
A band-aid fix is a code fix that solves the current issue. This refers to a fix that can be done fast but only works for a short amount of time and not a long term solution to the current problem.
In coding we often come accustomed to fixing the problem as fast as possible, not sometimes caring about the defect it can cause later down the line. The mentality of getting off your plate as soon as you can.
This leads to more problems as you grow as a developer. Not to mention that it might become a habit that could really hinder your growth as a developer.
You should try to avoid band-aid fix as soon as possible.
But if you can’t help using band-aid fix then I suggest asking your fellow / senior developers for advice regarding the issue you are having trouble with.
Most seniors already solved that problem before.
But if you don’t want to ask for advice. It's time to do research and expand your knowledge.
The more knowledge you have the less band-aid fix you will write.
Our job is to solve problems not create them.
About the Contributor
- Christian Dennis DulayView ProfileSoftware Engineer
Discuss this topic with an expert.
Related Topics
Boosting Website Performance with Lazy LoadingLazy loading is a web optimization technique that delays the loading of non-critical resources until they are needed. In this article, we take a deeper look at the benefits of lazy loading and how to implement this for your website.
Slow Websites Are Killing Your AI Conversions: The Hidden Costs of Poor PerformanceWhile companies race to adopt AI, they often overlook a fundamental and timeless factor: website performance.
Essential Guide to Core Web Vitals: Part 3Know your vitals: CLS