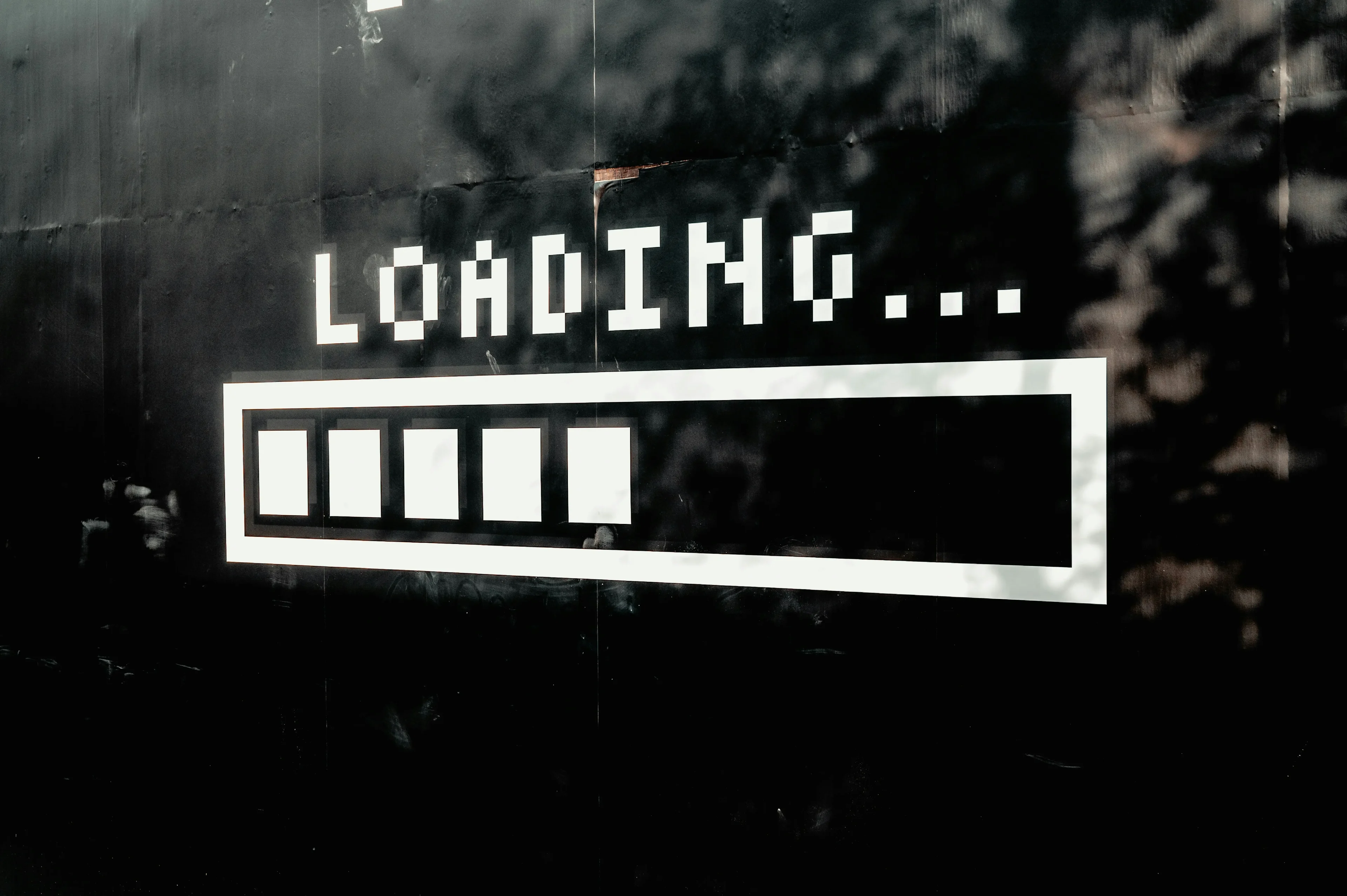
Boosting Website Performance with Lazy Loading
What Is Lazy Loading?
Lazy loading is a web optimization technique that delays the loading of non-critical resources until they are needed. Non-critical resources are usually images, videos, and scripts that won’t be immediately needed for rendering the page initially. Instead of loading all resources when a page first opens, lazy loading ensures that less critical resources are loaded only when the user needs it. This makes way for the critical resources, like the page’s stylesheet and resources that are immediately in view, to be prioritized and loaded earlier.
For example, an e-commerce website may include a carousel of recommended products with lots of images towards the bottom of the product page, so that users may see other recommendations related to the current product they are viewing. It may be the case that a user may not even scroll down far enough to see the product recommendation carousel, so loading all the resources needed for the carousel on page load may be unnecessary.
In this case, the browser should request for the necessary resources only when the user is about to scroll to the bottom of the page where the recommended products can be seen.
Why Is Lazy Loading Important?
In today’s fast-paced digital world, website performance is crucial. A slow-loading website can frustrate users, increase bounce rates, and hurt SEO rankings. Lazy loading helps improve performance by:
- Reducing initial load time: Only critical resources load first, shortening the Critical Rendering Path (CRP) and improving the First Contentful Paint (FCP) of a website.
- Saving bandwidth: When a web page is first rendered into view, resources like fonts, CSS, images, and scripts that are used by the whole page are downloaded by the user’s browser. When non-critical resources are lazy loaded, users only download images or videos they actually view, reducing unnecessary data usage.
- Enhancing user experience: Reducing CRP and FCP times means a faster website. Faster interactions lead to better engagement and satisfaction.
- Improving SEO rankings: Google considers page speed as a ranking factor, so optimizing loading time improves the website’s search visibility.
How to Implement Lazy Loading
Incorporating lazy loading into your website is simpler than you might think. One useful API that is commonly used for lazy loading is the Intersection Observer API which allows for knowing whether an observed element (in our earlier example, the product recommendation carousel) is entering the viewport.
Here are some common resources that are lazy loaded and ways to implement it:
1. Lazy Load Images with HTML
Modern browsers support lazy loading natively using the loading="lazy" attribute in images:
<img src="example.jpg" loading="lazy" alt="">
This tells the browser to load the image only when it enters the viewport. However, make sure that the lazy attribute is only used for non-LCP elements, as these elements need to be loaded as early as possible when the page is first rendered.
2. Lazy Load Videos
Videos are usually loaded with the <video> element, but videos hosted on other platforms may use <iframe> instead. For video elements, lazy loading can be achieved by specifying the preload attribute:
<video controls preload="none" poster="moviePoster.jpg">
<source src="movie.webm" type="video/webm">
<source src="movie.mp4" type="video/mp4">
</video>
By default, browsers preload some video data when a video element is present on the page. The preload=”none” tells the browser not to preload any video data. YouTube and other platforms offer an embed method via iframes that allows lazy loading:
<iframe loading="lazy" src="https://www.youtube.com/embed/your-video-id" frameborder="0" allowfullscreen></iframe>
This prevents the video from loading until the user scrolls near it.
3. Lazy Load JavaScript
Instead of loading large JavaScript files on initial page load, you can import them only when needed. A common use case for this would be when there is a modal on the page that typically wouldn’t be displayed until the user presses a button. In this case, the user interaction to watch out for would be the click—this will trigger the loading of the necessary JavaScript resource.
with JavaScript Dynamic Imports
if (userInteracts) {
import('./login-modal.js').then(module => {
module.default();
});
}
with NextJS Dynamic Import
import dynamic from 'next/dynamic'
const DynamicLoginModal = dynamic(() => import('../components/loginModal’), { loading: () => <p>Loading...</p>,})
export default function Home() {
. . .
return isLoginModalOpen && <DynamicLoginModal />
}
4. Lazy Load CSS
Stylesheets are typically render-blocking resources, which means that the browser will retrieve all necessary stylesheets before rendering a page. There is a way to tell the browser that it can load specific stylesheets at a later time by specifying the media attribute of the stylesheet:
<!-- the main stylesheet is render-blocking and is not lazy loaded as it contains the most critical styles in order for the page to be displayed properly -->
<link rel="stylesheet" href="styles.css" />
<!-- print.css for print media is not render-blocking and can be loaded at a later time -->
<link rel="stylesheet" href="print.css" media="print" />
<!-- mobile.css for mobile screens is not render-blocking on large screens and can be loaded at a later time -->
<link rel="stylesheet” href="mobile.css" media="screen and (max-width: 480px)" />
Conclusion
Lazy loading is a simple yet powerful technique that enhances website performance, reduces unnecessary data consumption, and improves user experience. By implementing lazy loading for images, videos, and scripts, you can ensure your site remains fast and efficient.
Need help pinpointing your website’s performance bottlenecks? Request a Good Web Vitals+ audit from us.
About the Contributor
- Riyana Elizabeth GuecoView ProfileFrontend Engineer
Discuss this topic with an expert.
Related Topics
Slow Websites Are Killing Your AI Conversions: The Hidden Costs of Poor PerformanceWhile companies race to adopt AI, they often overlook a fundamental and timeless factor: website performance.
Essential Guide to Core Web Vitals: Part 3Know your vitals: CLS
Essential Guide to Core Web Vitals: Part 2Know your vitals: INP